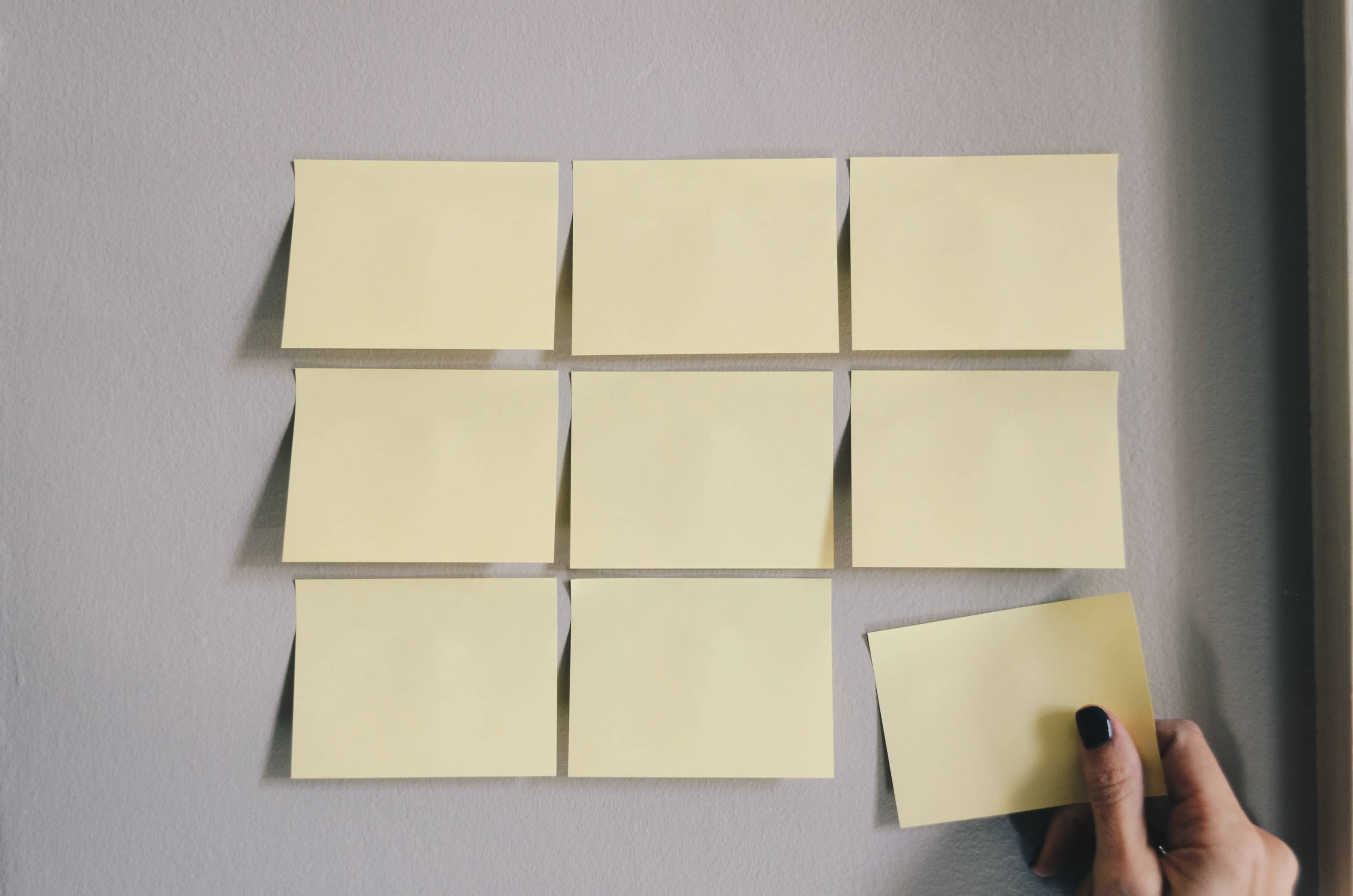
SaaS Security Checklist: How To Stop Worrying In 9 Steps

If you’ve ever built a SaaS, you know security can be intimidating. Serving people brings responsibilities that can feel overwhelming at times. SaaS applications are easy to target because they are easy to access for anyone with an Internet connection, which only brings in more anxiety. But it doesn’t have to be this way.
In the following article, we propose a SaaS security checklist to protect your revenues, the trust your users put into you, while avoiding costs a data breach would generate. At Onboardbase, we build a software vault for dev teams to secure their project environment configurations, so protecting our users’data is one of our core missions and we know what we’re talking about when it comes to securing a SaaS.
Without further ado, let’s see what you can do today to make your SaaS even greater.
8 Best Practices To Secure your SaaS
1. Strong Authentication And Authorization
Good auth is key to make sure your app understands who is who (authentication) and who can do what (authorization):
- Strong passwords - Strong passwords can’t be brute-forced in a realistic amount of time. If a password is only composed of numbers and is 5 characters long, you’d just need to try 59049 combinations to break it, so make sure passwords contain numbers, uppercase letters, and special characters, and are at least 8 characters long:
const password_generator = () => {
const length = 8
const charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!?,.;/:-=+{}"
let password = ""
const size = charset.length
for (let i = 0 ; i < length ; i++) {
password += charset.charAt(Math.floor(Math.random() * size));
}
return password;
}
- Fine-grained access roles - Disallow all file and endpoint accesses by default, unless the user is given the appropriate role to perform the actions. Each specific use case of your SaaS should have clear roles and scopes. For example, a bot user with the scope
message
should be able to query yourmessage
API, while regular users shouldn’t:
if(!(user.hasRole('bot') && user.hasScope('message'))){
return res.status(401).send('Unauthorized Access')
}
- Use third-party identity providers - Identity providers like Google or Facebook make SaaS auth more secure because they abstract away a lot of complexity like user verification, bot mitigation, two-factor authentication, or even adaptive authentication that use machine learning to prevent stolen identities that are hard to implement yourself.
const passport = require('passport')
const GoogleStrategy = require('passport-google-oidc')
passport.use(new GoogleStrategy(...), function verify() {...})
router.get('/login/google', passport.authenticate('google'))
2. Secure Your Environment Variables
Environment variables allow developers to store constants SaaS need to run, but whose value depend on the current environment―development, staging, production, etc. Environment variables also store sensitive information like API keys, to keep it separate from the application code which could leak to unauthorized users through Git repositories or public web folders.
- Don’t use env files - When env files aren’t properly secured, they can be accessed by anyone browsing the web. This is why you should always define environment variables whether through the web interface provided by your web hosting provider, or directly by specifying environment variables in your operating system’s .bashrc file:
export MY_API_KEY = <super secret api key>
- Use Onboardbase to manage your environment variables - Onboardbase makes it easy to store and use environment variables in any collaborative software project. Just try it by copy / pasting an environment variable and Onboardbase guides you through a quick 5-minute installation process:

After confirming your email you’re ready to use Onboardbase in your project:
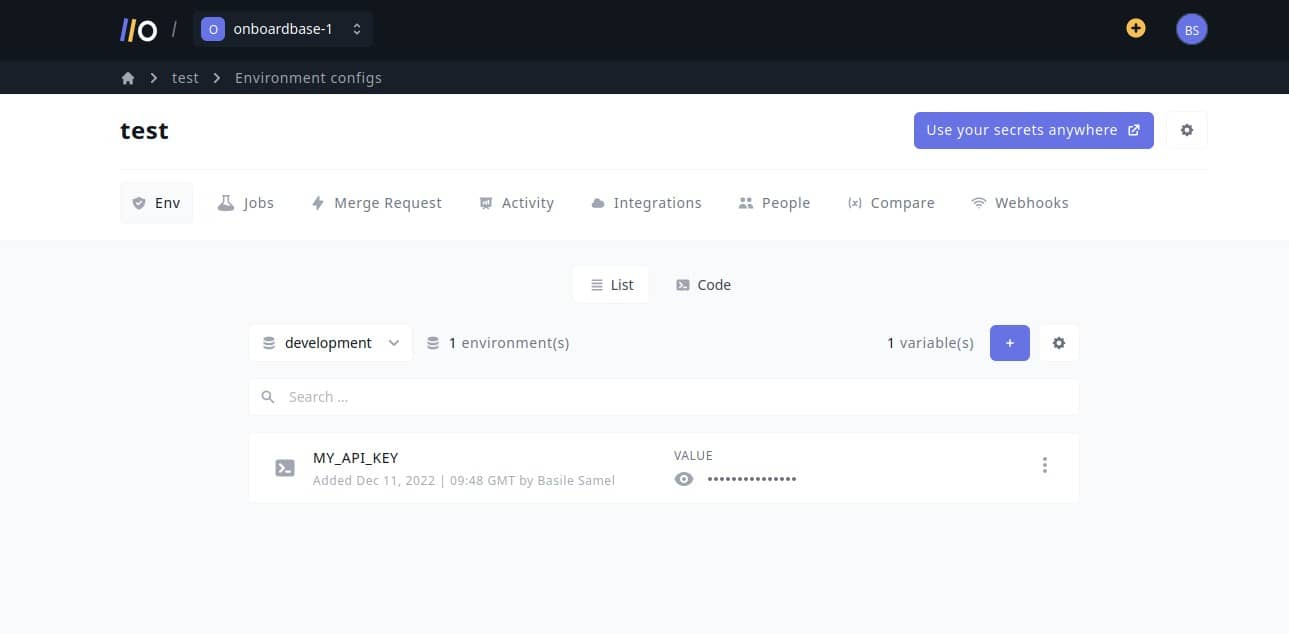
In your project, install Onboardbase’s CLI and set it up. For example, in a NodeJS project:
npm i -g @onboardbase/cli@latest
npm run onboardbase setup
And then inject environment variables at runtime using Onboardbase’s build
command:
onboardbase build --command="npm run start"
Onboardbase makes it easy to collaborate with your team securely, because you can choose who can use which environment variable using role-based access control features built-in. All data is stored and AES-256 encrypted in a centralized vault, and each query implements perfect forward secrecy using RSA, AES-GCM, and HTTPS.
3. Ditch Server-Side Rendering
Server-side rendering means running code on the server to generate the HTML for a web page when it is requested by a user: it’s an opportunity for an attacker to exploit a vulnerability in your code.
- Use static rendering - Static rendering is considered better for security because it allows you to pre-generate the HTML for a web page and store it on the server, which means that it can be served to users without running any code on the server. This reduces the attack surface of your web application and makes it less vulnerable to attacks. Look up frameworks like Astro.js or Hugo to get started faster.
- Use islands of interactivity for dynamic content - single page applications are harder to hack because you only have to take care of securing your API endpoints, but the Javascript payload can be detrimental to the user experience. One way to get away with the best of both worlds is to use a framework like Astro.JS or Remix to implement an islands architecture―small interactive Javascript components scattered in your app, instead of one big bundle that’s heavy to load.
- If you can’t remove SSR, test forms for XSS or SQL injection - Ditching server-side rendering is hard because it means refactoring your entire app, but you can do it iteratively by first separating your front-end and your back-end before switching to newer technologies. If the change isn’t worth it for you, just make sure to test your code for XSS vulnerabilities and SQL injections using things like input validation, request encoding, CSRF tokens, and web vulnerability scanners like OWASP ZAP.
4. Secure Your API Endpoints
34% of companies do not have an API security strategy, even with APIs running in a production environment. Because APIs can be accessed by anyone with an Internet connection, an unsafe API is a free ticket to your database.
Fortunately, APIs are relatively simple to secure when compared to front-end components: read our 8 API security best practices to learn how to protect your API endpoints without spending hours researching or hiring a developer.
5. Secure your database
There is no SaaS without data. Securing your database is vital to your business.
- Automated backup - Something can happen to your database―bad migration, service shortage, sabotage―so you might as well be ready for it. Backing up your data regularly is standard practice. While most admin dashboards provide a way to regularly backup databases, you can also do it yourself with cron jobs. With a MySQL database, for example, you can use the
mysqldump
command to store your database as a file:
mysqldump -u [user name] –p [password] [options] [database_name] [tablename] > [dumpfilename.sql]
- Encrypted sensitive data - Some parts of your database need to be encrypted to prevent leaks from affecting your users. This is the case for passwords for example: you never want password to readable for anyone but your corresponding user, including your database admins. Here is how to encrypt your data with the AES-256 algorithm:
import aes256 from 'aes256'
const key = 'a passphrase'
const my_password = 'a secret password'
const encryptedPassword = aes256.encrypt(key, my_password)
const decryptedPassword = aes256.decrypt(key, encryptedPassword)
- Prevent SQL injections - Pick a database ORM to handle safe database access. Proper encoding, input validation, and character pre-processing prevent users from trying to pass SQL commands to your database queries through form inputs or API calls. If you’re not sure about what you need to do―not everybody is a database expert―it’s best to just abstract away your database queries with a trusted open-source library or a third-party services.
const { Sequelize } = require('sequelize')
const sequelize = new Sequelize({...})
const User = sequelize.define('User', {...});
const users = User.findAll({
where: {
username: <input_username>
}
})
6. Implement a CI/CD pipeline
Implementing a Continuous Integration / Continuous Delivery pipeline has never been easier: you can get started for free with Github Actions in minutes, connect your Git repository, and that’s it.
Devops helps identifying security issues quicker, even before they are released in the wild if done properly. But you need to take care of two points to make CI/CD worth it:
- Automated testing - Without a testing suite, your pipeline loses a lot of effectiveness: no breaking change should be shipped to your production environment. Not only does it help identifying vulnerabilities early in the development process, but they will also be a lot easier and cheaper to fix with unit tests ready.
steps:
- uses: actions/checkout@v3
- name: Use Node.js
uses: actions/setup-node@v3
with:
node-version: '12.x'
- run: npm install
- run: npm test
- run: npm run build --if-present
- Automated environment configuration - With Onboardbase, you can even automate environment configuration from within your pipeline to prevent manual human errors. Onboardbase injects environment variables at build time without needing to define them in your pipeline:
- run: npm run onboardbase build --command="npm run start"
7. Prepare For DDoS Attacks
5.4 million Distributed Denial of Service (DDoS) attacks were orchestrated in the first semester of 2021 alone―it’s the most common type of cyber attack where websites of any size can be a target. As a SaaS business, DDoS attacks are probably the most disarming type of attack because your web servers are flooded with requests, making it impossible for you to login or do anything if you don’t have the right infrastructure in place.
Work with your internet service provider (ISP) to implement additional measures to protect against DDoS attacks. Your ISP may be able to provide additional bandwidth or other resources to help mitigate the attack.
- Use load balancing - A load balance splits incoming traffic among multiple web servers to prevent bottlenecks. You can easily implement one with a web server configuration. For example, in a Caddy server configuration file:
reverse_proxy server1:80 server2:80 server3:80
-
Serve your website from a CDN - A content delivery network acts like a load balancer, but on a global scale to prevent localized network attacks. With a solution like Cloudflare’s CDN, your SaaS will not only load faster because the web servers will be closer to your users, but you’ll also benefit from their built-in DDoS protection. There is no coding involved, you just need to register your domain name with Cloudflare for free.
-
Use a web application firewall - Use a web application firewall (WAF) to block malicious traffic with custom security rules. For example, the following Caddy configuration prevents users from hotlinking your website’s images, which would result in higher traffic load:
https://onboardbase.com {
root /var/www/onboardbase-website/
rewrite {
if {>Referer} not ""
if {>Referer} not "https://onboardbase.com"
to /hotlink
}
status 403 /hotlink
}
- Rate limiting - Rate limiting prevents users from spamming your server’s endpoints. For example, a regular user shouldn’t be able to crawl your whole website under a minute, except if it’s a Google Search bot. A rate limit can prevent such behavior easily. Rate limiting is best implemented in a reverse proxy like Nginx or Caddy rather than directly in your server code, for performance reasons.
8. Always Be Monitoring
If a security breach happens and you have no way to notice it, a long time can pass before anything can done about it. It took Toyota 5 years to notice a data leak, so nobody is protected from it: monitoring your SaaS for unusual activity patterns can be a life saver! Monitoring, like CI/CD, helps you identify threats early, prevent breaches, and decrease your response time.
-
Install product analytics - Tools like Cloudflare or Google Analytics help identify traffic spikes as well as their origin. If you know where malicious activities originate from, you can blacklist IP addresses and quickly get rid of attackers.
-
Use server logs - All web servers offer the ability to monitor incoming and outgoing traffic. You just need to enable the setting. Logs contain information such as the IP address of a user, the corresponding URL, and the HTTP parameters. With this, you can block any suspicious activity as it happens.
-
Monitor secret usage and leaks - Onboardbase helps teams detect secrets(environment variables) spilled in their codebase with a single
scan
command:
onboardbase scan
Combined with the usage dashboard, you’ll know exactly when a secret leaks and who is responsible:
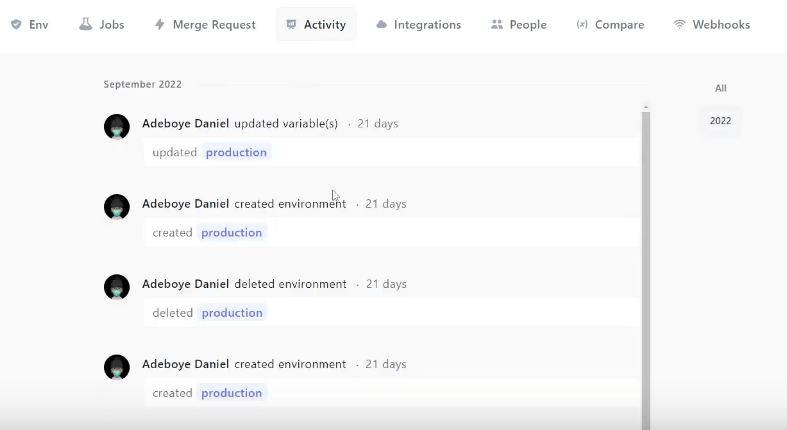
Protect Your Users, Subscribe To Onboardbase
Onboardbase makes the lives of SaaS developers easier by streamlining how they manage environment variable configurations. It’s the only tool you’ll need to secure your SaaS deploys throughout the whole software development lifecycle. A dashboard centralizes SaaS configs for the whole team, and a command-line interface is available to integrate environment variables in any workflow. Get started for free in a minute, it’s super easy.
Subscribe to our newsletter
The latest news, articles, features and resources of Onboardbase, sent to your inbox weekly